How to disable and lock Gutenberg blocks
Gutenberg makes it easy to build content with blocks, but sometimes, you need control over which blocks are available. Maybe you’re working on a client site and want to prevent them from using certain blocks. Or perhaps you’re streamlining the editing experience by removing unnecessary options.
In this guide, we’ll explore different ways to disable Gutenberg blocks, including:
- Using the WordPress user interface (UI) to hide blocks in the inserter
- Locking blocks to prevent them from being moved or deleted
- Enforcing block restrictions with PHP, including role-based access
That said, we won’t be covering block visibility (showing/hiding content based on conditions) or disabling specific block settings like text or background colors, which is handled in theme.json
. However, we will discuss block locking since it’s closely related to disabling blocks.
All the methods in this guide work without plugins and apply to any block-based theme. Let’s get started!
Disabling blocks with the WordPress UI
Removing unnecessary blocks helps streamline the editing experience and can slightly improve backend performance, as disabled blocks are not loaded into memory.
Any user can disable blocks from the Preferences menu in the block editor. You can do this by clicking the three-dot Settings (⋮) menu in the top-right corner opens the editor preferences. Then, under the Blocks tab, users can uncheck any block to remove it from the block inserter.
For example, you can disable the Quote block by simply unchecking its box, as shown below.
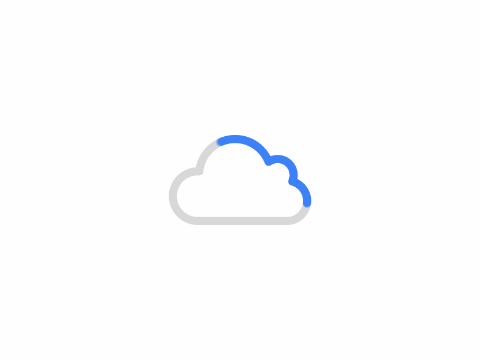
If you want to go further, you can disable an entire block category. For instance, unchecking the Text category will remove all text-related blocks from the inserter, ensuring they are no longer available for use. This can be helpful for streamlining the editor and preventing users from accessing unnecessary blocks.
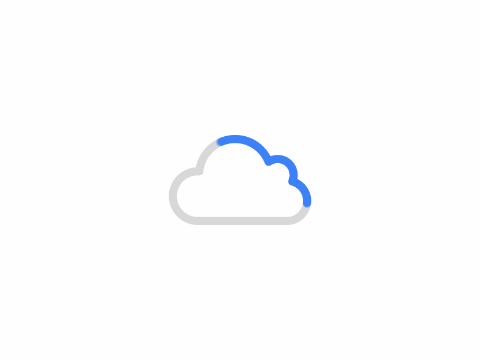
Disabling blocks with PHP
There are two fundamental and very distinct approaches to allowing or preventing a block from being used with WordPress. Depending on your needs you may choose either to allow or deny a block from being available in the Inserter.
Both approaches can be implemented using PHP or JavaScript, each with its own advantages and drawbacks. PHP is generally simpler when allow-listing blocks, while JavaScript is often more efficient for deny-listing.
We are using PHP for all of our examples to demonstrate various use cases.
Allow-listing blocks
To allow only specific blocks in the inserter, use the following filter. This ensures that only the designated blocks are available for all users:
add_filter('allowed_block_types_all', 'allowed_block_types_all_users', 10, 2 );
function allowed_block_types_all_users( $allowed_blocks, $block_editor_context ) {
return array(
'core/paragraph',
'core/heading',
'core/image',
'core/cover',
'core/list',
'core/list-item'
);
}
This code should be added to the functions.php
file of a child theme to prevent changes from being lost when the theme is updated.
When using this method, ensure that all necessary child blocks are included. For example, if you allow the core/list
block, you must also include core/list-item
to prevent errors.
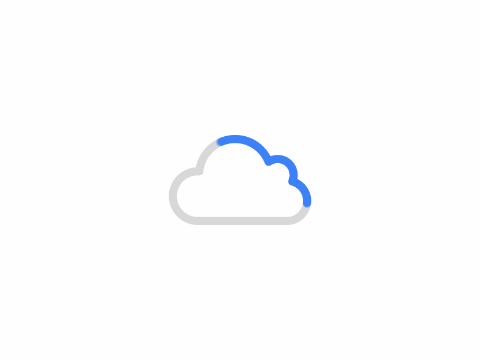
The allowed_block_types_all
filter gives developers control over the blocks available in the inserter. It accepts two parameters:
$allowed_block_types
— An array or boolean defining the permitted blocks (default: true).$block_editor_context
— Provides information about the current block editor state, including the post being edited.
Allowing specific blocks for contributors and authors
The following code restricts the available blocks for users without the publish_pages
capability (contributors and authors):
add_filter('allowed_block_types_all', 'allowed_block_types_for_non_admins', 10, 2);
function allowed_block_types_for_non_admins($allowed_blocks, $block_editor_context) {
// Apply restrictions if the user does not have the 'publish_pages' capability
if (!current_user_can('publish_pages')) {
// Define the allowed blocks for users without 'publish_pages' capability
$allowed_blocks = array(
'core/paragraph',
'core/heading',
'core/image',
'core/cover',
'core/list',
'core/list-item'
);
}
return $allowed_blocks;
}
In the above example, Contributors and Authors can only use paragraph, heading, image, cover, and list blocks.
Allowing blocks for specific post types and users
The following code adds the Shortcode block to the inserter when editing a page but keeps it unavailable for other post types:
add_filter('allowed_block_types_all', 'allowed_block_types', 25, 2);
function allowed_block_types($allowed_blocks, $editor_context) {
$allowed_blocks = array(
'core/paragraph',
'core/heading',
'core/image',
'core/cover',
'core/list',
'core/list-item'
);
// Check if the editor context has a post object and if its type is 'page'
if (!empty($editor_context->post) && 'page' === $editor_context->post->post_type) {
$allowed_blocks[] = 'core/shortcode';
}
return $allowed_blocks;
}
Keep in mind that since contributors add authors can’t create or modify pages the result will appear only in a post.
All users will only see six blocks but administrators and editors will also see the shortcode block available only for a page.
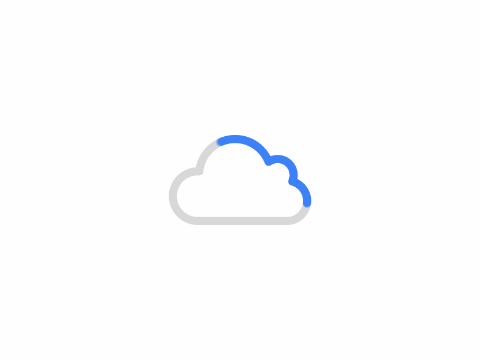
In our example, the impact this has on contributors and authors is null as, by default, they cannot add new pages. However, using a Role Manager plugin could alter that capability.
Allowing blocks based on Post ID
If there are instances where you wish to allow a set of blocks only for certain posts here is how you can do it:
add_filter('allowed_block_types_all', 'allowed_block_types', 10, 2);
function allowed_block_types($allowed_blocks, $editor_context) {
// Check if the editor context has a post object
if (!empty($editor_context->post)) {
$post_id = $editor_context->post->ID;
// Define allowed blocks for specific post IDs
$allowed_blocks_by_post = array(
2 => array('core/paragraph', 'core/heading', 'core/image'),
3 => array('core/paragraph', 'core/heading', 'core/image')
);
// Check if the current post ID has a defined allowed blocks array
if (array_key_exists($post_id, $allowed_blocks_by_post)) {
return $allowed_blocks_by_post[$post_id];
}
}
return $allowed_blocks;
}
In this example, only paragraph, heading, and image blocks will be available for post IDs 2 and 3.
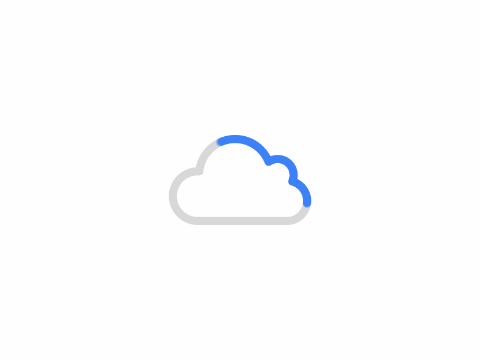
This is fine for a small set of post IDs. But if you have a dynamic situation where pages or posts are added continuously, then consider filtering on taxonomies and custom fields.
Deny-listing blocks
Allow-listing, by implication, is a form of deny-listing as blocks not available are denied. But you can take a reverse approach if you prefer to allow most blocks except for a few. In this example, the heading and cover blocks are no longer available to any user.
add_filter('allowed_block_types_all', 'deny_blocks');
function deny_blocks($allowed_blocks) {
// Get all registered blocks
$blocks = WP_Block_Type_Registry::get_instance()->get_all_registered();
// Disable two specific blocks
unset($blocks['core/heading']);
unset($blocks['core/cover']);
return array_keys($blocks);
}
Essentially, we find all registered blocks and then remove the heading and cover blocks.
Be careful if you think you can unset any block with this method. If a block — core or otherwise — is registered with JavaScript you must unregister it with JavaScript.
Deny-listing entire block categories
If you want to remove entire categories of blocks, such as Widgets, Embeds, or Theme blocks, use this approach:
add_filter('allowed_block_types_all', 'disable_blocks_by_categories', 10, 2);
function disable_blocks_by_categories($allowed_blocks, $editor_context) {
// Get all registered blocks
$registered_blocks = WP_Block_Type_Registry::get_instance()->get_all_registered();
// Specify the categories to disable
$categories_to_disable = array('widgets', 'embed', 'theme');
// Initialize an array to hold allowed block names
$allowed_block_names = array();
// Loop through registered blocks
foreach ($registered_blocks as $block_name => $block_type) {
// Check if the block has categories defined
if (isset($block_type->category)) {
// If the block's category is NOT in the disabled list, allow it
if (!in_array($block_type->category, $categories_to_disable, true)) {
$allowed_block_names[] = $block_name;
}
} else {
// If the block has no category defined, allow it by default
$allowed_block_names[] = $block_name;
}
}
return $allowed_block_names;
}
This approach filters out entire categories of blocks, simplifying the block editor experience.
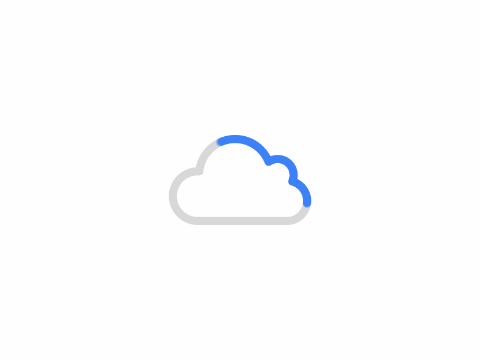
Locking blocks with the WordPress UI
Locking a block prevents it from being moved or deleted while still allowing content edits. Any user can lock or unlock a block at any time using the Lock option in the block toolbar.
To either lock or unlock a block, click the three-dot Settings (⋮) on the block, click Lock and then selecting the Lock all option automatically enables both Prevent movement and Prevent removal, but these options can also be applied separately.
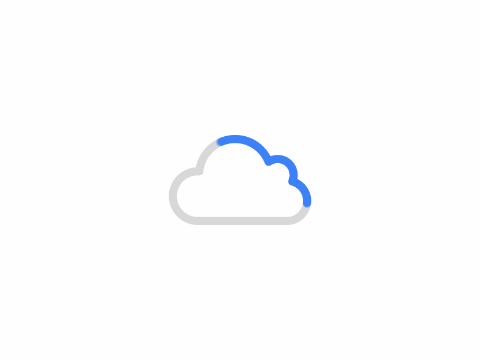
It’s important to know that even when a block is locked, users can still change its content and style unless further restrictions are applied.
Preventing styling changes is not possible through the lock feature alone. To restrict block styling, modifications must be made in the theme.json
file.
For blocks containing nested elements, there is an additional option to lock only the parent block or lock all inner blocks as well. This ensures that grouped elements remain structured while allowing controlled edits within them.
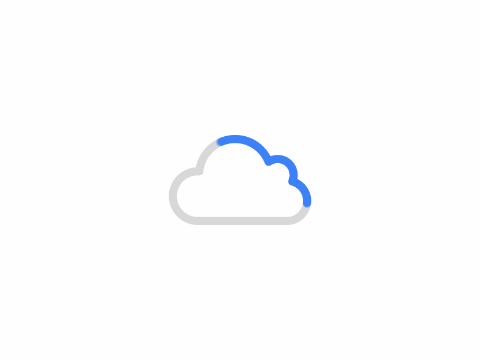
Locking blocks with PHP
While the WordPress UI provides basic block locking, it does not enforce restrictions site-wide. Any user with editor access can unlock a block, making it easy to override locked content. To permanently restrict block locking, PHP is the best solution.
With PHP, you can completely remove the ability to lock and unlock blocks, ensuring that no user can bypass restrictions. This is how WordPress functioned before the release of WordPress 5.9, when block locking was introduced.
Block locking is useful in many scenarios, particularly when maintaining structured content. By enforcing block restrictions with PHP, you can:
- Preserve design integrity by preventing users from modifying key blocks.
- Prevent accidental editing that could break layouts.
- Streamline content creation by reducing unnecessary options.
- Ensure consistency in patterns and templates, especially for client projects.
Removing block locking functionality for all users
The following PHP snippet disables block locking entirely, preventing any user from locking or unlocking blocks:
add_filter('block_editor_settings_all', 'example_disable_block_locking', 10, 2);
function example_disable_block_locking($settings, $context) {
$settings['canLockBlocks'] = false;
return $settings;
}
With this applied, the block locking feature is completely removed from the block editor. Users will not see the lock options, and no one regardless of role will be able to lock or unlock blocks.
For users hosting their site with Kinsta, making changes to theme files is easy and secure using SFTP, which is enabled by default for all WordPress sites.
Restricting block locking based on user roles
Instead of completely removing block locking, you may want to restrict who can lock and unlock blocks. The following PHP snippet allows only administrators and editors to modify block locks, while authors and contributors cannot unlock any block set by an admin or editor.
add_filter('block_editor_settings_all', 'example_disable_block', 10, 2);
function example_disable_block ($settings, $context ) {
if (
isset( $context->post ) &&
'post' === $context->post->post_type &&
! current_user_can( 'edit_theme_options' )
) {
$settings['canLockBlocks'] = false;
$settings['codeEditingEnabled'] = false;
}
return $settings;
}
This approach limits block control to users with the edit_theme_options
capability, typically administrators and editors. Authors and contributors will not be able to unlock blocks set by higher-level users.
Additionally, access to the Code Editor is disabled, preventing users from manually modifying block markup to bypass restrictions. This ensures that locked blocks remain unchanged, even by users with coding knowledge.
Summary
Choosing whether to allow or deny blocks — or a combination of both — depends on your specific needs. You might want to restrict certain blocks for a cleaner editing experience, enforce design consistency, or control access based on user roles.
Speaking of user roles, capabilities can be modified to further customize how blocks are managed. This opens up even more possibilities beyond what we’ve covered here.
Keep in mind that WordPress evolves over time. Future updates could introduce new ways to manage blocks or modify existing functionality, so staying up to date with WordPress development is important to ensure your approach remains effective.
Are you looking for a secure and developer-friendly hosting solution? Kinsta makes it easy to manage your WordPress files, including editing theme files via SFTP, ensuring safe and seamless customizations without risking site stability.
The post How to disable and lock Gutenberg blocks appeared first on Kinsta®.
共有 0 条评论