面向对象的细胞实现
书名:代码本色:用编程模拟自然系统
作者:Daniel Shiffman
译者:周晗彬
ISBN:978-7-115-36947-5
第7章目录
7.8 面向对象的细胞实现
在后面的工作中,我们将为CA系统添加一些扩展特性,其中很多都涉及细胞的多个属性,比如:让细胞记住最近的10个状态;在CA系统中加入运动和物理学特性,让细胞能在屏幕中移动,在每一帧里动态地改变它的邻居。请问,这些特性该如何实现?
1、Cell类
- 一个细胞对象有哪些属性?
在本例中,每个细胞都有一个位置和大小,当然还有状态。
class Cell {
float x,y; 位置和尺寸
float w;
int state; 细胞的状态是什么?
- 在非面向对象版本中,我们用两个不同的二维数组存放当前和下一代的状态。用对象表示细胞后,每个细胞可以同时保存这两个状态。因此,我们希望细胞能记住之前的状态(在计算新状态之前)。
int previous; 之前一代的细胞状态是什么? - 这让我们能将更多状态变化信息可视化。比如,我们可以对状态发生变化细胞赋予不同的颜色
2、示例
代码7-3 面向对象的生命游戏
GOL gol;
void setup() {
size(640, 360);
gol = new GOL();
}
void draw() {
background(255);
gol.generate();
gol.display();
}
// reset board when mouse is pressed
void mousePressed() {
gol.init();
}
- Cell.pde
class Cell {
float x, y;
float w;
int state;
int previous;
Cell(float x_, float y_, float w_) {
x = x_;
y = y_;
w = w_;
state = int(random(2));
previous = state;
}
void savePrevious() {
previous = state;
}
void newState(int s) {
state = s;
}
void display() {
if (previous == 0 && state == 1) fill(0,0,255);
else if (state == 1) fill(0);
else if (previous == 1 && state == 0) fill(255,0,0);
else fill(255);
stroke(0);
rect(x, y, w, w);
}
}
- GOL.pde
class GOL {
int w = 8;
int columns, rows;
// Game of life board
Cell[][] board;
GOL() {
// Initialize rows, columns and set-up arrays
columns = width/w;
rows = height/w;
board = new Cell[columns][rows];
init();
}
void init() {
for (int i = 0; i < columns; i++) {
for (int j = 0; j < rows; j++) {
board[i][j] = new Cell(i*w, j*w, w);
}
}
}
// The process of creating the new generation
void generate() {
for ( int i = 0; i < columns;i++) {
for ( int j = 0; j < rows;j++) {
board[i][j].savePrevious();
}
}
// Loop through every spot in our 2D array and check spots neighbors
for (int x = 0; x < columns; x++) {
for (int y = 0; y < rows; y++) {
// Add up all the states in a 3x3 surrounding grid
int neighbors = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
neighbors += board[(x+i+columns)%columns][(y+j+rows)%rows].previous;
}
}
// A little trick to subtract the current cell's state since
// we added it in the above loop
neighbors -= board[x][y].previous;
// Rules of Life
if ((board[x][y].state == 1) && (neighbors < 2)) board[x][y].newState(0); // Loneliness
else if ((board[x][y].state == 1) && (neighbors > 3)) board[x][y].newState(0); // Overpopulation
else if ((board[x][y].state == 0) && (neighbors == 3)) board[x][y].newState(1); // Reproduction
// else do nothing!
}
}
}
// This is the easy part, just draw the cells, fill 255 for '1', fill 0 for '0'
void display() {
for ( int i = 0; i < columns;i++) {
for ( int j = 0; j < rows;j++) {
board[i][j].display();
}
}
}
}
3、运行结果
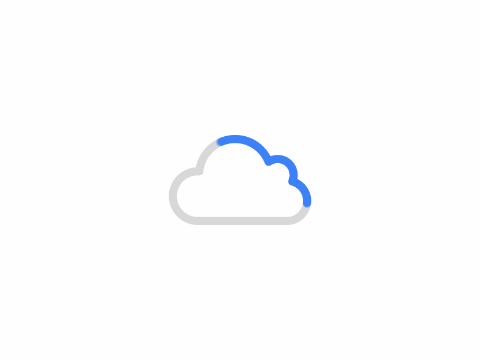
共有 0 条评论