How your plugin can customize the WordPress Command Palette
The release of WordPress 6.3 introduced the Command Palette, a feature that offers quick access to actions frequently used by those who edit content or themes within the CMS’s admin interface.
The menu-like Command Palette allows users to filter available tasks using a simple search interface and select options to help navigate the editor’s UI, toggle preferences, transform styles, manipulate blocks, and even begin creating new posts and pages.
Also available is a JavaScript-enabled API that allows developers to add functionality to the Command Palette. For example, the creator of a WordPress plugin that generates Web forms might add a Command Palette entry that whisks users off to a page that shows the results of form submissions.
The developer of a plugin that uses many shortcodes might link an entry in the Command Palette to a pop-up “cheat sheet” to remind users how to use those codes.
The possibilities are endless, and we’re giving you a taste of how the API works to inspire you to use the Command Palette in your next WordPress plugin project.
WordPress Command Palette basics
You launch the Comand Palette by using the keyboard shortcut Cmd + k
(Mac) or Ctl + k
(Windows and Linux) or clicking on the Title field at the top of the Post Editor or Site Editor:
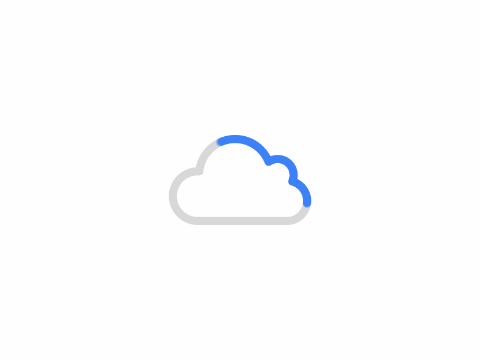
The top of the Palette includes a search field that filters entries as you type. You can select entries using a mouse or using the keyboard alone.
A partial list of commands available out of the box in the Palette includes:
- Edit Template (when editing a page)
- Back to page (after editing its template)
- Reset template
- Reset template part
- Reset styles to default
- Delete template
- Delete template part
- Toggle settings sidebar
- Toggle block inspector
- Toggle spotlight mode
- Toggle distraction free
- Toggle top toolbar
- Open code editor
- Exit code editor
- Toggle list view
- Toggle fullscreen mode
- Editor preferences
- Keyboard shortcuts
- Show/hide block breadcrumbs
- Customize CSS
- Style revisions
- Open styles
- Reset styles
- View site
- View templates
- View template parts
- Open navigation menus
- Rename Pattern
- Duplicate Pattern
- Manage all custom patterns
And, of course, developers can add their own to enhance their WordPress applications. Let’s jump into the process!
What you’ll need to get started
The Comand Palette API is supported by JavaScript packages you will add to your projects using npm
, the Node Package Manager. You’ll need an installation of WordPress (local or remote) that you can access via the terminal to execute commands on the command line.
To get things rolling, we created a WordPress plugin that will be home to the code that modifies the Command Palette. The plugin does little more than create a custom post type that we call Products. (Everything you need to know to get this far with a rudimentary plugin is available in our guide to creating custom post types.)
When our Product Pages plugin is activated, we gain a Dashboard menu entry for creating and browsing Product posts:
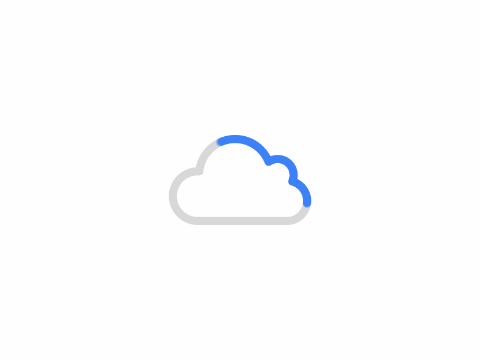
Our plugin does not have any unique assistance from the WordPress Command Palette. For example, the default functionality of the Command Palette provides shortcuts to add new WordPress posts and pages:
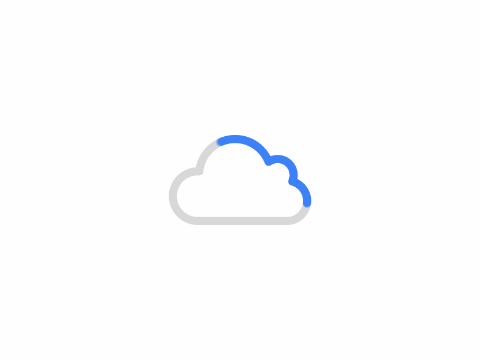
However, the Command Palette knows nothing about creating our Product pages. We are adding that functionality below.
Adding a custom command to the Command Palette
Right now, our entire Product Pages plugin consists of a single PHP file that we have named products.php
and placed in wp-content/plugins/products
. Other than enable the Products post type, it doesn’t do anything yet. We’ll come back to this file after we’ve set up the JavaScript-powered API for the Command Palette.
Installing the API dependencies
We start by creating a generic package.json
file in the products
directory by moving to that directory in the terminal and running the command:
npm init
It’s not critical how you respond to the init
prompts, but you will want to provide a name and description for your application. (We used products as the name and Product Pages as the description.)
Now that you have a skeletal package.json
file, open it in your favorite code editor and add the following lines somewhere in the body, perhaps after the description
line:
"scripts": {
"build": "wp-scripts build --env mode=production"
},
Still in the terminal, we can add the dependency for the WordPress Scripts — @wordpress/scripts
— package:
npm install @wordpress/scrips --save
That will add the following lines to our plugin’s package.json
file:
"dependencies": {
"@wordpress/scripts": "^30.5.1"
}
Now, we can execute npm run build
, but the process expects an index.js
file (even if empty) in our plugin’s src
directory. We’ll create those and run the build using the following commands in the terminal:
mkdir src
touch src/index.js
npm run build
That will create a build
directory for our production JavaScript (index.js
). Like the one in src
, that file will be blank right now. Also, in the build
directory, you should find the file index.asset.php
.
If things appear to be built correctly, let’s go ahead and add the remaining dependencies by running these commands in the terminal within the plugin’s root directory:
npm install @wordpress/commands @wordpress/plugins @wordpress/icons --save
Take a look in the package.json
file now, and the dependencies block should look something like this:
"dependencies": {
"@wordpress/commands": "^1.12.0",
"@wordpress/icons": "^10.12.0",
"@wordpress/plugins": "^7.12.0",
"@wordpress/scripts": "^30.5.1"
}
The newly added WordPress Commands package interfaces with the Command Palette, the Plugins package is savvy about WordPress plugins, and the Icons package allows you to select and display members of a pre-built library of images.
Adding a Command Palette hook and enqueuing our script
Now that we have our resources in place, we need to add code to our empty src/index.js
file that will actually hook into the Command Palette. Open the file in your editor and add this code:
import { useCommand } from '@wordpress/commands';
import { registerPlugin } from '@wordpress/plugins';
import { plus } from '@wordpress/icons';
const AddProductCommand = () => {
useCommand( {
name: 'add-product',
label: 'Add new product',
icon: plus,
callback: ( { close } ) => {
document.location.href = 'post-new.php?post_type=kinsta_product';
close();
},
context: 'block-editor',
} );
return null;
}
registerPlugin( 'products', { render: AddProductCommand } );
export default AddProductCommand;
Some notes on the above code:
- A single icon (the plus figure) is imported from the Icons package.
useComand()
is the hook that registers the command.- The label Add new product is the text that will become an entry in the Command Palette.
- The
callback
(what happens when a user clicks on Add new product) simply opens the WordPress new post PHP file with a query string that specifies a Product post. (Until now, the only thing our plugin could do.)
With that saved, it’s time for a final build:
npm run build
After building, build/index.js
will contain our production JavaScript. The final step is to enqueue the script in WordPress. We do that by adding the following code to the bottom of our products.php
file (the simple PHP file that established the plugin and defines the Product post type):
// Enqueue assets.
add_action( 'enqueue_block_editor_assets', 'enqueue_block_editor_assets' );
/**
* Enqueue assets.
*
* @return void
*/
function enqueue_block_editor_assets() {
wp_enqueue_script(
'products',
plugins_url( 'build/index.js', __FILE__ ),
array(),
'1.0',
true
);
}
With that complete, we’ll return to the WordPress editor and activate the Command Palette. We should see Add new product in the list after typing the appropriate search text:
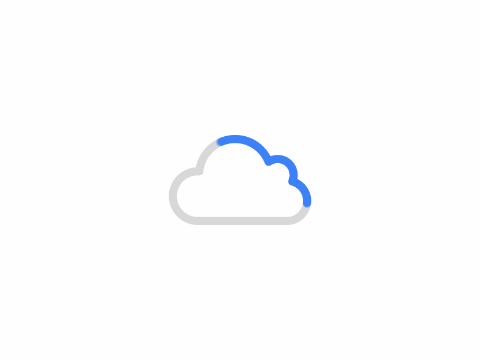
If we select Add new product from the Palette, WordPress will open the Post Editor on the path reserved for our new Product content type.
Summary
The Command Palette API opens up a flexible way to integrate your application with one of the more interesting features of modern WordPress. We’d be interested in learning if you have taken advantage of the API and what you achieved.
Developers like you can count on Kinsta to provide powerful, Managed WordPress Hosting solutions that support performant and scalable projects.
Try Kinsta today to see how we can help you build top-tier WordPress sites!
The post How your plugin can customize the WordPress Command Palette appeared first on Kinsta®.
共有 0 条评论