Redis object caching for WordPress: the complete installation guide
WordPress powers a huge chunk of the web, but its popularity highlights the challenge of maintaining optimal performance. One powerful solution for improving WordPress performance is Redis object caching. Using this in-memory key-value database as a cache reduces the number of queries sent to a site’s primary database.
This guide shows you how to install and work with Redis object caching for your WordPress website. For Kinsta customers, the process is particularly straightforward.
Understanding object caching
When you load a WordPress page, your server typically needs to make multiple database queries to fetch the content, settings, and other data it needs to display the page. Each query takes time, and as your site grows, these small delays can add up to noticeable slowdowns.
Object caching helps by storing the results of these database queries in memory. The cache stores the queries you use often and waits until you need them.
Object caching can transform how your WordPress site handles data retrieval and processing, and the impact extends beyond simple speed improvements. When your site experiences sudden traffic spikes, such as during a successful marketing campaign or after a viral social media post, Redis can act as a buffer between your visitors and your database.
Rather than each visitor triggering new database queries, Redis will serve cached data from memory. This can let a site handle significantly more concurrent users across your entire site without performance degradation.
For an e-commerce site on Black Friday, Redis-cached product information could reduce the database load and let that site handle more traffic volume without requiring additional server resources. This efficiency translates directly into hosting cost savings, as it can serve more visitors with the existing infrastructure.
How WordPress uses its database
Understanding the way WordPress interacts with its database can help to explain why caching becomes crucial as a site grows. Consider what happens when someone visits your site’s home page. Unless it’s responding with the results of a page cache, WordPress orchestrates a complex symphony of database queries to build a dynamic page.
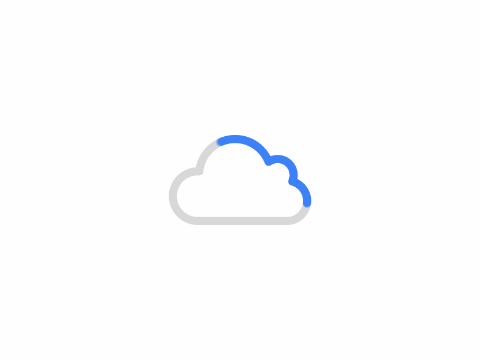
Let’s walk through a typical home page load: first, WordPress queries the wp_options
table to fetch your site’s settings, theme configuration, and active plugins.
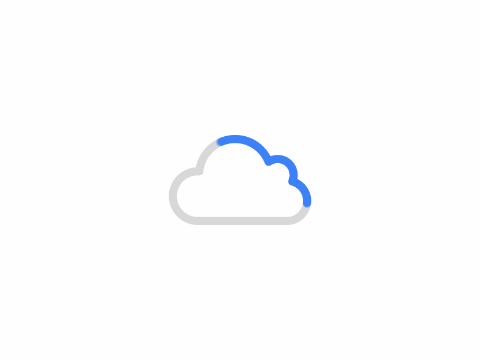
If you have extra widgets, Blocks, or elements in your sidebar, this will trigger additional queries. For example, a Recent Posts section will need post data, categories need term counts, and any search functionality needs to build an index.
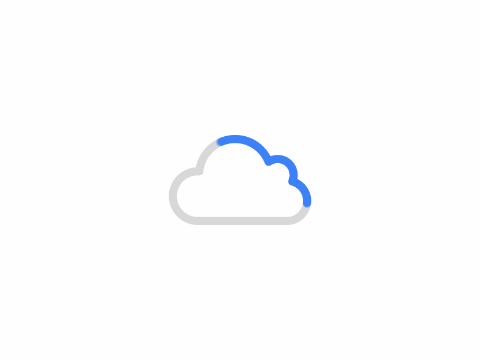
If you’re using a page builder plugin or an otherwise complex theme, these queries will multiply significantly. The complexity compounds with dynamic content. Take a common blog setup where posts display author information, categories, tags, and related posts:
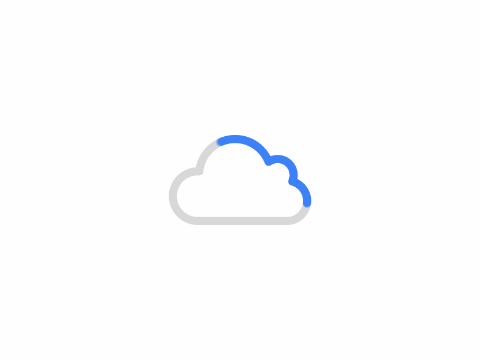
Each post preview on your home page requires WordPress to join data across multiple tables. It pulls the core content from wp_posts
, grabs author details from wp_users
, and collects metadata from wp_postmeta
. A home page displaying just ten post previews might execute dozens of separate database queries.
The bottlenecks within the WordPress database
This database architecture also reveals common bottlenecks that impact performance. Custom post types, while powerful for organizing content, often need to rely on wp_postmeta
for storing additional fields.
Some websites — think of an online store or real estate directory — might make hundreds of queries per page load just to display each product or property. Each must display individual details such as square footage, quantity, pricing, bedrooms, variations, and more as separate metadata entries.
The wp_options
table can become another bottleneck. This is because it will store the settings for any plugins that offer them.
The impact becomes more pronounced when you factor in concurrent visitors. Each user will trigger their own set of queries, and WordPress will carry out independent processing for each of them. During traffic spikes, this processing can create a bottleneck that slows down an entire site.
These database interactions make caching invaluable. If you implement Redis object caching properly, it can intercept these repeated queries and store the results in memory. Instead of executing multiple joins and metadata queries for each visitor, WordPress can retrieve the pre-processed data directly from Redis. The result is that it can often reduce dozens of database queries down to a single cache lookup.
Popular choices for WordPress object caching
When it comes to object caching solutions for WordPress, several options are available. Not every host will support all options, which means you need to ensure your choice of object cache can achieve what you need.
Memcached is one of the oldest and most widely used caching systems. It’s a distributed memory caching system that’s simple and effective. It has a lot of support thanks to its longevity and is generally lightweight when it comes to resource usage. With good support and documentation, Memcached is a popular solution for object caching at all levels.
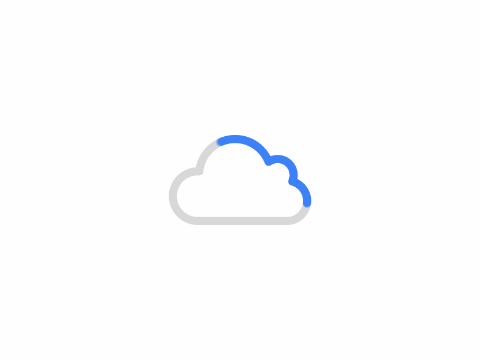
Given this focus on ease of use, more complex scenarios might not be suitable for this simple key-value store. It also doesn’t offer “persistent” storage, which means it will clear by the time the next page loads.
Couchbase can offer a more complex solution that combines document database capabilities, typical key-value storage functionality, and built-in clustering. The latter technology automates data grouping for better performance — similar to how Windows Disk Defragmenter works to improve that operating system’s disk performance.
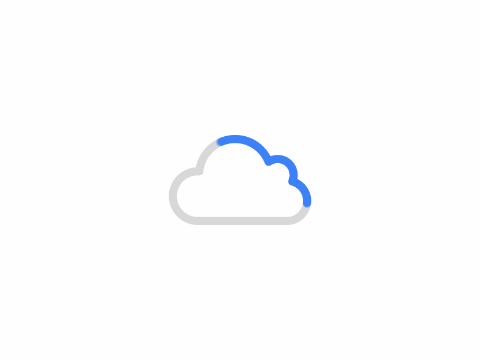
However, Couchbase’s key-value storage is secondary to its document-driven architecture. This could be a problem if you need fewer query limitations and greater accuracy in data validation and consistency.
Why Redis stands out for WordPress
For WordPress, Redis offers several advantages over the direct competition. Unlike Memcached, Redis supports complex data structures such as lists, sets, and sorted sets. This aligns well with WordPress’s data organization needs and gives you a way to scale up towards greater and more complex setups.
When it comes to using these different structures, Redis’s “atomic operation” is crucial. In a nutshell, this uses a concept of transactions and groups various commands together to run at once. The actual functionality is more complex than this, but atomic operations typically ensure data consistency — which is crucial for any WordPress website.
There are two more benefits of using Redis with WordPress:
- Persistence. Redis can persist data to disk. This provides better data durability compared to an in-memory solution.
- Better memory management. Redis offers more sophisticated memory management options than other caching tools. This can give you better control over your object cache’s behavior.
Redis has applications that go beyond object caching, but for WordPress, the unique makeup of the database solution means its key-value storage is almost an ideal partner.
The relationship between WordPress and Redis
WordPress includes its own object caching functionality through the WP_Object_Cache
function. At its core, this acts as an intermediary layer between your site’s code and the database using standardized functions to manage cached data.
When a plugin or theme requests data, WordPress first checks if the data exists in the object cache using these built-in functions. For example, here’s code that retrieves a user’s comment count:
function get_user_comment_count($user_id) {
// Generate a unique cache key
$cache_key = 'user_comment_count_' . $user_id;
// Try to get the value from cache first
$comment_count = wp_cache_get($cache_key, 'user-stats');
// If not in cache, query the database
if (false === $comment_count) {
global $wpdb;
$comment_count = $wpdb->get_var(
$wpdb->prepare(
"SELECT COUNT(*) FROM $wpdb->comments WHERE user_id = %d",
$user_id
)
);
// Store the result in cache for future requests
wp_cache_set($cache_key, $comment_count, 'user-stats', 3600); // Cache for 1 hour
}
return $comment_count;
}
When properly configured with Redis, the function intercepts database requests and checks if the required data exists in the Redis cache before WordPress makes a database query.
And the integration extends beyond simple key-value storage. Redis’s ability to handle complex data structures mirrors the WordPress hierarchical content organization. For instance, when WordPress needs to retrieve a complex query result, such as all child pages of a parent page with their associated metadata, Redis stores this entire data structure as a single cache entry.
This integration could substantially improve performance. Redis stores all data in memory, which means access times are in microseconds rather than the milliseconds typically required for database queries. This doesn’t seem valuable, but for sites with heavy database usage, this difference could net page load times that are two or three times faster.
WordPress’s object cache also supports advanced Redis functionality through additional configuration. For example, you can implement cache tags for more granular cache management:
function get_category_posts($category_id) {
$cache_key = 'category_posts_' . $category_id;
$posts = wp_cache_get($cache_key, 'category-posts');
if (false === $posts) {
$posts = get_posts(array(
'category' => $category_id,
'posts_per_page' => 10
));
wp_cache_set(
$cache_key,
$posts,
'category-posts',
3600,
array(
'tags' => array(
'category_' . $category_id,
'front_page_content'
)
)
);
}
return $posts;
}
// Later, when a post in this category updates:
wp_cache_delete_by_tag('category_' . $category_id);
This relationship between WordPress and Redis creates a powerful caching system that intelligently manages data persistence while maintaining data consistency. The WP_Object_Cache
function ensures that all your plugins and themes can benefit from Redis caching without requiring direct implementation. Also, advanced functionality in Redis provides the flexibility you need for complex WordPress installations.
Kinsta customers can install Redis in less than 5 minutes
Consider a typical scenario: your WooCommerce store slows down with increased traffic. At many web hosts, implementing Redis involves accessing the server, manual installation, configuring security settings, and careful testing. This could easily be a day of technical work — and more if you encounter errors. Kinsta’s Redis implementation transforms this process entirely.
You have the option to add Redis object caching with a few clicks in the MyKinsta dashboard for $100 USD a month. Customers can navigate to WordPress sites > sitename > Add-ons > Redis caching (or WordPress sites > sitename > Caching > Redis) and click the Enable button:
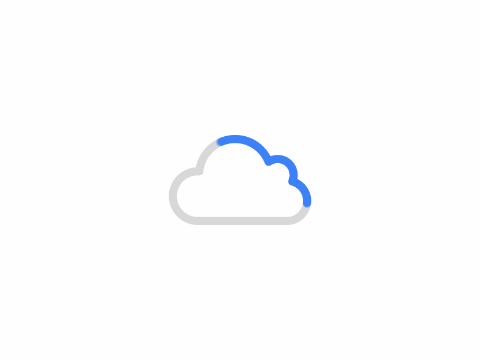
Kinsta’s integration can have a big impact on your site and its performance:
- It applies an optimal configuration for WordPress websites. This includes adjusting cache expiration times — for instances where cart abandonment issues could be problematic. Expiration time optimization is a common issue that can plague improperly configured Redis installations.
- The Redis integration runs silently in the background. For you, this is good news as you can continue with managing your site while benefiting from the performance object caching will provide.
- You have flexibility when it comes to monitoring your object cache and have deep integration with Kinsta’s functionality and architecture.
The integration with other tools within MyKinsta is a huge benefit, as Redis becomes part of your overall caching strategy. Monitoring the impact of the performance is also a key facet you must consider.
How to implement Redis for your Kinsta website
The initial setup for Redis object caching with your Kinsta-hosted WordPress site is fast. When you enable the add-on, Kinsta automatically installs and configures the Redis Object Cache plugin. This cuts down the need for further setup and configuration. You also have the flexibility to use a different connection plugin if you wish, although you will need to disable the Redis Object Cache plugin from within WordPress to do so, click the Deactivate link in the plugin’s group within the WordPress admin dashboard:
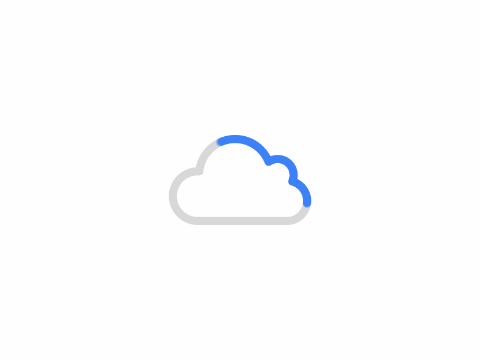
Much of managing your Redis installation will happen on your WordPress website through your plugin’s settings. This includes clearing the cache. The Kinsta MU plugin adds this option to the WordPress toolbar:
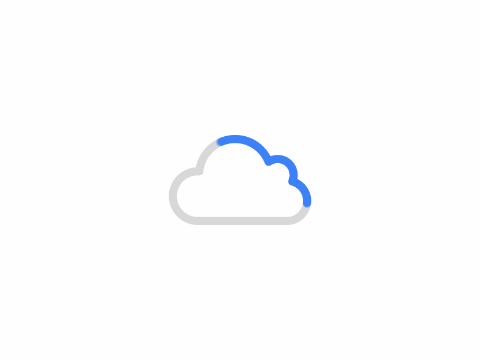
However, there are a few ways you can clear the Redis cache beyond WordPress. For example, the WordPress sites > sitename > Caching > Server Caching screen within MyKinsta will allow you to achieve this:
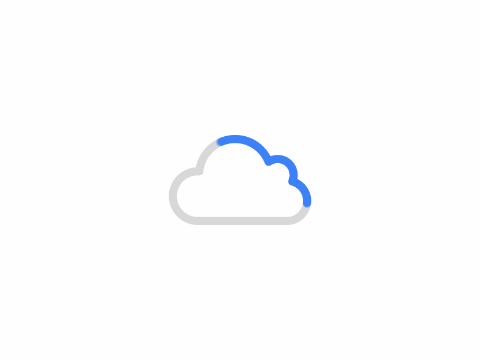
This option clears all of the caches your site uses, as does the alternative approaches using Secure Shell (SSH) and the WP-CLI.
How to install Redis on some other WordPress web hosts
While Redis is a popular way to set up an object cache, not every host will offer access or integration. This means you may need to get your hands dirty with code on your server.
Each web host will have a different approach to doing this — some may not even give you the root access you need. However, the typical steps will include preparing the server, installing Redis, and then configuring WordPress to use it.
Server preparation and installation
Installing Redis requires a properly configured server environment. For some WordPress hosts, this may mean you choose a suitable plan. It’s likely that you won’t be able to do this on typical shared hosting or even managed tiers. A virtual private server (VPS) will be the starting point for your efforts, but dedicated cloud hosting will be ideal.
Regardless, your PHP installation will need the phpredis extension. Installing this lets Redis work with PHP — essential for also working with WordPress. You will need to use specific compilation flags and configuration options, of which there are many.
On Ubuntu systems, install the required components with:
sudo apt-get update
sudo apt install redis server
Once the installation process completes, run sudo service redis status
to check that Redis is running in the way you’d expect. You may also want to run redis-cli --version
to check that the installation completes as you’d expect too.
Once you have Redis running on your server, you can install the phpredis
extension:
sudo apt-get install php-redis
sudo phpenmod redis
This is all you need to do to install Redis, but you still need to configure it for your server and your available resources.
Redis configuration
The Redis server configuration file will need your attention before you let it begin to work on your site. The first task is to understand whether WordPress and Redis work on the same server. Typically, this will be the case, so you need to bind the localhost address (127.0.0.1
).
You can choose any editor for the job of accessing your Redis configuration file, but nano is perfect and will be available on almost every server instance you find:
sudo nano /etc/redis/redis.conf
In the majority of cases, you can find the right line and uncomment it before you save your changes:
bind 127.0.0.1 ::1 # listens on loopback IPv4 and IPv6
You may want to make further changes to this configuration file. Here’s an optimal setup for WordPress sites:
maxmemory 256mb
maxmemory-policy allkeys-lru
appendonly yes
appendfsync everysec
save 900 1
save 300 10
save 60 10000
Each configuration choice serves a specific purpose:
- The
maxmemory
setting of 256MB provides a good starting point for most WordPress installations. This setting prevents Redis from consuming excess system memory while maintaining enough cache space to improve performance significantly. - The
allkeys-lru maxmemory-policy
ensures the most frequently accessed content stays in cache. Some sites benefit fromvolatile-lru
instead, especially when caching session data alongside regular content. - The
appendonly
andappendfsync
settings manage Redis’s persistence behavior. While Redis serves primarily as a cache, maintaining persistence prevents complete cache losses during server restarts. Theeverysec
setting balances performance with data safety.
The save
directives control when Redis creates point-in-time snapshots of the dataset. The example configuration tells Redis to save:
- Every 15 minutes after one change.
- Every five minutes after 10 changes.
- Every minute after 10,000 changes.
These persistence settings will help maintain cache efficiency while protecting against data loss.
Configuring Redis security and testing changes
You should also look into security here. For example, you can set up password authentication using the requirepass
command and even rename “dangerous” commands. The Redis access control list (ACL) puts limitations on certain destructive commands, and you should look over the whole list to see if any may affect you.
Once you complete all of these steps, it’s a good idea to test your Redis server’s performance. The Redis CLI offers several benchmark commands for this purpose:
redis-cli --latency
redis-cli info | grep used_memory_human
redis-cli info | grep connected_clients
In short, they establish baseline performance metrics for ongoing monitoring and should be part of your regular maintenance workflow.
Configuring WordPress
Once Redis is running on your server, WordPress needs to be configured to use it as an object cache. The configuration typically includes specifying the Redis connection details, such as the host, port, and any authentication credentials.
You could manually add the appropriate object cache drop-in file to your wp-content
directory, although installing a dedicated Redis object cache plugin can be the best way to achieve this. The only one we recommend here is the Redis Object Cache plugin mentioned above, as Kinsta doesn’t support many caching plugins due to its own functionality. The Redis Object Cache plugin is more of a helper for connecting WordPress to the key-value store.
Redis management beyond the installation
Typical Redis object cache installations provide access to the Redis CLI. At Kinsta, this extends all the way through your development workflow, such as with staging environments and DevKinsta.
Fundamental monitoring
This command line interface hands you powerful capabilities to connect to your Redis instance and gain immediate insight into how your cache operates. For instance, you can reveal cached data patterns, analyze memory usage, and execute maintenance tasks in real-time.
For basic monitoring, there are a few essential commands to note:
redis-cli INFO stats # View cache hits and misses
redis-cli INFO memory # Check memory utilization
redis-cli MONITOR # Watch live cache operations
The MONITOR
command streams cache operations in real time, which shows exactly how WordPress interacts with Redis. This visibility helps you to identify cache patterns and optimization opportunities. The SLOWLOG
command identifies problematic queries:
redis-cli SLOWLOG GET 10 # View the 10 slowest recent operations
redis-cli SLOWLOG RESET # Clear the slow log for fresh monitoring
You have options that extend much further into what Redis can offer.
Deeper Redis monitoring commands
A simple way to rein in resources is to monitor Redis’s connection limits. It’s an excellent way to prevent resource exhaustion:
redis-cli CLIENT LIST | wc -l # Count active connections
redis-cli CONFIG GET maxclients # Check maximum allowed connections
WordPress uses Redis as a way to speed up the read access for its database. The cache entries are persistent and can always be cached again in the future. To support that, Redis supports “eviction policies” for keys it stores.
However, this can bring drawbacks in the form of memory pressure. A low “hit ratio” — which compares the total number of operations to those on existing keys — is evidence of that, so tracking the following metrics could be vital:
redis-cli INFO stats | grep evicted_keys
redis-cli INFO stats | grep hit_rate
If you find your database does suffer from memory pressure, you can choose to increase the available memory, optimize any key expiration policies, and implement selective caching strategies. The exact approach you take will depend on your site and the pressure your memory is under.
Using a GUI with Redis
There’s much more to discover with Redis commands and using the CLI, although it won’t be an appropriate tool for some. This is where the Redis Insight app could be useful.
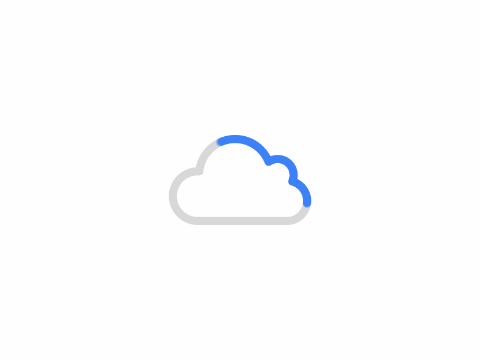
This gives you a GUI to view your Redis object cache without the need for a terminal, server access, or command line work. As you would work with a tool such as TablePlus or SequelAce to view your WordPress database, an app such as Redis Insight is quick to set up and could streamline your workflow.
Common Redis challenges and solutions
Most of the time, your Redis installation will work without further maintenance. However, some Redis implementations can present challenges that will demand your attention. For example, you might see a warning within MyKinsta that WordPress can’t detect a suitable connection plugin.
This appears when you choose to use a plugin other than Redis Object Cache and is safe to ignore under the vast majority of circumstances. However, note that optimally running Redis relies on a suitable connection plugin.
For example, you might not see the right metrics when analyzing within the Kinsta APM Tool (or other Kinsta analytics). This should be something you can counteract and resolve if you choose to build a custom Redis instance with Kinsta.
It’s also a good idea to understand your limitations with Kinsta’s Redis integration. For instance, you may see various errors where you use a non-typical WordPress installation type. Using a Bedrock installation is a common reason for errors and is something the Kinsta Support team can help you resolve.
Summary
Redis object caching delivers powerful performance improvements for WordPress sites through efficient data storage and retrieval. Success lies in proper implementation, regular monitoring, and maintenance. Utilizing Kinsta’s managed solution lets you work within these principles to ensure optimal site performance.
Each stage of Redis implementation builds on the previous one. First, start with proper server configuration. Then, integrate with WordPress integration. Finally, performance gains can be maintained through regular monitoring and optimization. This approach will help you create a robust caching infrastructure that grows with your site, with Kinsta at the base.
Do you have any challenges that Kinsta’s implementation for Redis object caching solves? We welcome your insights in the comments section below!
The post Redis object caching for WordPress: the complete installation guide appeared first on Kinsta®.
共有 0 条评论