WordPress geolocation made simple: a developer’s guide
Modern web applications demand a high level of intelligent, location-aware functionality. E-commerce platforms need to calculate international shipping rates and content sites have to deliver region-specific news. Geolocation can transform static WordPress sites into dynamic, personalized experiences. However, it can be a struggle to implement this functionality, especially if you don’t have the right hosting provider.
This comprehensive guide explores how to implement powerful geolocation features in WordPress. It also features a special focus on Kinsta’s built-in capabilities that eliminate some of the most common headaches of adding geolocation to your site.
Understanding geotargeting and geolocation
Location-aware applications center on two key terms: geotargeting and geolocation. They’re related, but each serves a distinct purpose in your WordPress toolkit:
- Geolocation pinpoints your visitors’ locations.
- Geotargeting delivers specific content or experiences based on that data.
Netflix demonstrates this effectively: As you travel abroad, the streaming service determines your current country through geolocation, then adjusts your content library through geotargeting to match regional licensing agreements. This combination creates a seamless experience while maintaining compliance with international media rights.
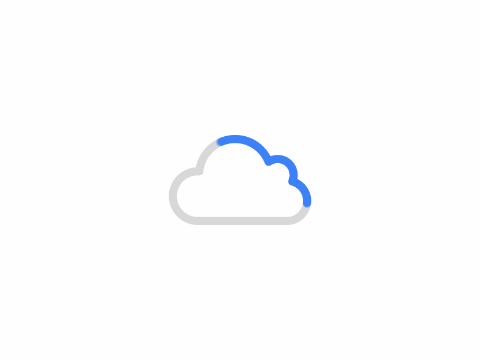
There are two primary methods for detecting the visitor’s location:
- IP-based location detection relies on databases that map IP addresses to geographical regions. It works universally without requiring user permission. Most server-side geolocation solutions use this, including Kinsta’s built-in capabilities.
- GPS-based detection accesses a device’s location hardware through browser APIs. It provides precise coordinates but requires explicit user consent. This functionality is behind “Near Me” location searches or weather applications that need exact positioning.
Geolocation and geotargeting work together, but within the former, there are multiple technologies that can work for a given use case. Neither is “better” than the other — there are benefits that transcend individual adaptations.
The business case for geolocation
Implementing geolocation for any website can deliver tangible benefits to both your business and users. First, personalization drives engagement. If your site can personalize the experience for each user, more of them may do business with you.
Amazon is one example of how you can reap the rewards. Its shopping site can show shipping times for each product based on your location:
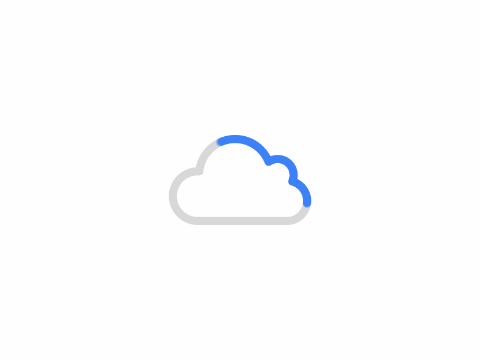
Depending on your location, you can also see relevant times for Amazon Prime events, such as sports games:
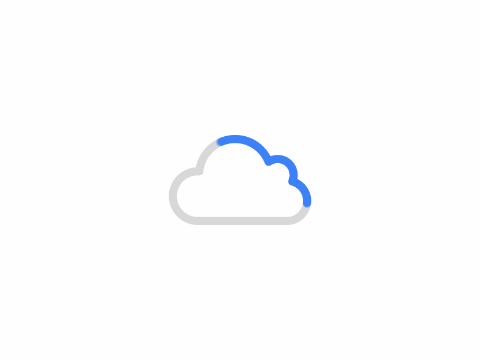
You can see this across the web, such as how weather sites automatically display your local forecast. Regardless of the implementation, personalization reduces friction in the user experience, and can significantly improve conversion rates.
Regulatory compliance also depends on location awareness. The GDPR in Europe, the CCPA in California, and many more regions mandate specific requirements for handling user data. Proper geolocation implementation helps you meet such requirements for each visitor.
Finally, localized content builds trust. Research demonstrates that users complete purchases more often when prices appear in their local currency and shipping information remains clear. For example, a Common Sense Advisory study shows that three-quarters of consumers prefer buying products in their native language.
How to integrate geolocation with WordPress
WordPress’ flexible architecture means there are multiple approaches if you wish to add location awareness to your site. Your choice of website hosting, use of plugins, coding knowledge, and more will all factor into your preferred approach. However, working with the data itself can happen in a few ways.
Working with geographical data in WordPress
While WordPress core includes a lot of fundamental functionality, this doesn’t include built-in geolocation. There’s nothing within WordPress core that records location data, although it does support storing and processing location data in several ways.
The WordPress database
For example, the WordPress database can store coordinates and location data using custom fields or dedicated location tables.
It can also handle location-based taxonomies. This works well for store locators or real estate listing services that run a custom location database. WordPress doesn’t store any geolocation data by default though — it’s simply that the ability is there to do so.
The WordPress REST API
The WordPress REST API also supports working with geolocation data, although it doesn’t include dedicated endpoints. You would need to create your own endpoint extensions to build location-aware applications that communicate with external services or mobile apps. Here’s an example:
add_action('rest_api_init', function() {
// Create a custom namespace for your geolocation endpoints
register_rest_route('your-site-geo/v1', '/location', [
'methods' => 'GET',
'callback' => 'handle_location_request',
'permission_callback' => function() {
return true;
}
]);
});
function handle_location_request($request) {
// Access geolocation data (example using Kinsta's implementation)
$location = [
'country' => $_SERVER['GEOIP_COUNTRY_CODE'] ?? null,
'city' => $_SERVER['GEOIP_CITY'] ?? null,
'latitude' => $_SERVER['GEOIP_LATITUDE'] ?? null,
'longitude' => $_SERVER['GEOIP_LONGITUDE'] ?? null
];
return new WP_REST_Response($location, 200);
}
This creates a new endpoint at /wp-json/your-site-geo/v1/location
that returns location data for any API consumers.
The code uses your-site-geo
as a custom namespace. Your namespace should be specific to your needs to avoid conflicts with other plugins or custom code. It’s sound to follow the WordPress namespace guidelines:
- Use a vendor or package-specific prefix to avoid conflicts.
- Include a version number (such as
v1
). - Keep endpoints specific and focused.
You can also register location data for existing endpoints:
add_action('rest_api_init', function() {
register_rest_field('post', 'location_data', [
'get_callback' => function($post) {
return get_post_meta($post['id'], 'location_data', true);
},
'update_callback' => function($value, $post) {
update_post_meta($post->ID, 'location_data', $value);
},
'schema' => [
'type' => 'object',
'properties' => [
'latitude' => ['type' => 'number'],
'longitude' => ['type' => 'number'],
'country' => ['type' => 'string'],
'city' => ['type' => 'string']
]
]
]);
});
In many cases, the REST API will be where you turn to first to build in geolocation functionality, which means having this flexibility will be a boon.
Custom post types
You might also use custom post types in WordPress. If so, the new content types you create can include location metadata. This lets you organize content using geographic information, without the need for complex database modifications.
The first step is to register the post type with WordPress:
register_post_type('store_location', [
'public' => true,
'label' => 'Store Locations',
'supports' => [
'title',
'editor',
'Custom-fields' // Enables custom fields and meta
]
]);
You should also create a custom meta box for storing the coordinates you collect, and generate the HTML for it:
<?php
add_action('add_meta_boxes', function() {
add_meta_box(
'store_location_coordinates',
'Store Coordinates',
'render_location_meta_box',
'Store_location' // The post type where this meta box should appear
);
});
function render_location_meta_box($post) {
// Get existing coordinates if they exist
$latitude = get_post_meta($post->ID, 'latitude', true);
$longitude = get_post_meta($post->ID, 'longitude', true);
// Output the form fields
?>
<label>Latitude:
<input type="number"
step="any"
name="latitude"
value="<?php echo esc_attr($latitude); ?>">
</label>
<label>Longitude:
<input type="number"
step="any"
name="longitude"
value="<?php echo esc_attr($longitude); ?>">
</label>
<?php
}
The key part is to save the location data once you publish or otherwise save the post in WordPress:
add_action('save_post_store_location', function($post_id) {
// Verify if this is an autosave
if (defined('DOING_AUTOSAVE') && DOING_AUTOSAVE) {
return;
}
// Save latitude if it exists
if (isset($_POST['latitude'])) {
update_post_meta(
$post_id,
'latitude',
sanitize_text_field($_POST['latitude'])
);
}
// Save longitude if it exists
if (isset($_POST['longitude'])) {
update_post_meta(
$post_id,
'longitude',
sanitize_text_field($_POST['longitude'])
);
}
});
Building a function to grab any nearby locations could take a number of forms. Here’s a rough example of one that (in theory) fetches the latitude and longitude of a location:
function get_nearby_locations($lat, $lng, $radius = 10) {
$locations = get_posts([
'post_type' => 'store_location',
'posts_per_page' => -1
]);
$nearby = array_filter($locations, function($location) use ($lat, $lng, $radius) {
$store_lat = get_post_meta($location->ID, 'latitude', true);
$store_lng = get_post_meta($location->ID, 'longitude', true);
return calculate_distance($lat, $lng, $store_lat, $store_lng) <= $radius;
});
return $nearby;
}
Using any of these choices depends on your specific needs. For instance, use custom fields for simple location metadata or extend the REST API for headless implementations. For location-centric content, custom post types could be best.
The plugin approach to geolocation
WordPress supports plugins for almost everything, and there are numerous solutions for adding location awareness to your site, too. For example, If-So Geolocation or Geolocation IP Detection have great reviews and ratings, regular updates, and healthy support systems:
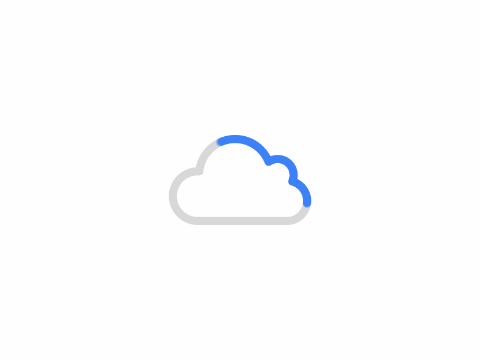
These provide lots of typical functionality in a simple package that also uses a familiar format. Plugins can provide a number of benefits for when you need to implement geolocation:
- You get rapid deployment and provide quick implementation without the need for custom code.
- There’s no maintenance on your end, as this is something the plugin developer handles (along with updates).
- There’s often community support and documentation in place to assist if you need it.
For the type of development you’ll want to carry out, a plugin might not be the best option. For starters, you rely on the quality of that plugin for your functionality. If you offer custom WordPress products, this might not be a partnership you want either. Each plugin will come with its own location database implementation, and the quality here could vary just as much as other features.
Plugins are excellent for end users or site owners who need to add location awareness to an existing site. However, the potential for conflicts with other plugins, the additional server load, and limited flexibility for custom implementations mean you may want a more robust option.
Real-world applications of WordPress geolocation
You don’t have to look far to find examples of WordPress products using geolocation in its codebase. For instance, WooCommerce includes built-in geolocation for tax calculations and shipping rules.
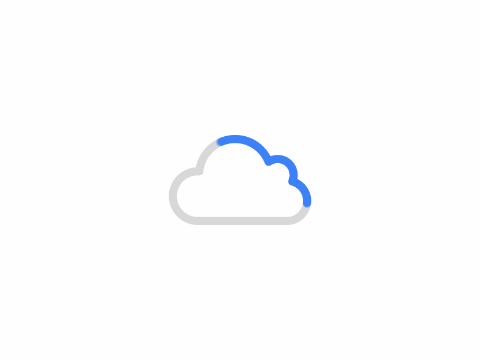
It uses MaxMind’s GeoIP2 database to detect customer locations automatically, which helps to ensure accurate pricing and delivery options from the first page load.
Other plugins will also offer support for geolocation. A form plugin like Gravity Forms’ Gravity Geolocation add-on is a typical use case. It lets users enter their location into a form, which Gravity Forms will then assign a set of coordinates and other relevant data.
This proves particularly valuable for lead generation and service request forms. More importantly, you have the flexibility to adapt the need for location awareness to your specific use case.
The Kinsta advantage: native geolocation capabilities
If you’re a Kinsta customer, you don’t need extravagant and inelegant solutions for implementing geolocation. The MyKinsta dashboard supports robust location detection without the overhead of additional WordPress plugins.
Understanding Kinsta’s geolocation system
Kinsta’s geolocation implementation leverages two powerful technologies:
- NGINX’s native geolocation module provides efficient, server-level location detection.
- MaxMind’s GeoIP2 database ensures accurate and up-to-date location mapping.
This integration within the MyKinsta dashboard offers several advantages over a plugin- or code-based solution:
- Because the location detection happens at the server level, there’s a minimal performance impact relative to other approaches.
- Kinsta carries out regular maintenance of the functionality, which means you get current and up-to-date geolocation.
- You have reliable location awareness and detection for your site without the need for JavaScript or browser permissions.
- There’s also additional integration with Kinsta’s Edge Caching system.
The Kinsta IP Geolocation tool includes integration with WordPress and can give you premiere functionality that performs well and is adaptable. This is thanks to how Kinsta exposes the location information and passes it onto the rest of your stack.
Available location data
WordPress uses PHP, and the IP Geolocation tool passes some location-centric information to the $_SERVER
PHP variable.
There are a number of endpoints and variables available to you that return different sets of data:
// Basic geographical data
$country_code = $_SERVER['GEOIP_COUNTRY_CODE']; // Two-letter country code (such as "US" or "GB")
$country_name = $_SERVER['GEOIP_COUNTRY_NAME']; // Full country name
$region = $_SERVER['GEOIP_REGION']; // State/province code
$city = $_SERVER['GEOIP_CITY']; // City name
$postal_code = $_SERVER['GEOIP_POSTAL_CODE']; // Local postal or ZIP code
// Precise location data
$latitude = $_SERVER['GEOIP_LATITUDE']; // Decimal latitude
$longitude = $_SERVER['GEOIP_LONGITUDE']; // Decimal longitude
There are more variables to use, such as various country and city code formats. Regardless, all of the exposed variables give you a way to code custom PHP based on the functionality of Kinsta’s IP Geolocation.
Implementing geolocation through MyKinsta
As with much of the functionality within MyKinsta, setting up geolocation through the dashboard is straightforward. The first port of call is the Tools screen for any of your sites:
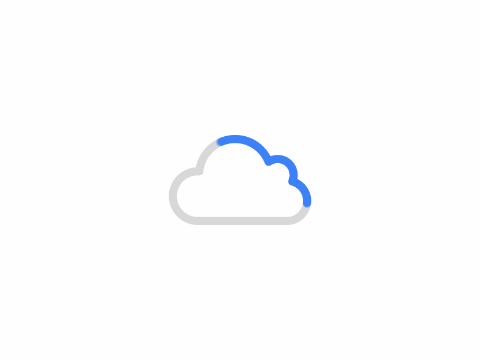
Clicking the Enable button on the Geolocation card will display a modal dialog box that asks you to choose to set geolocation for either the country level or country and city.
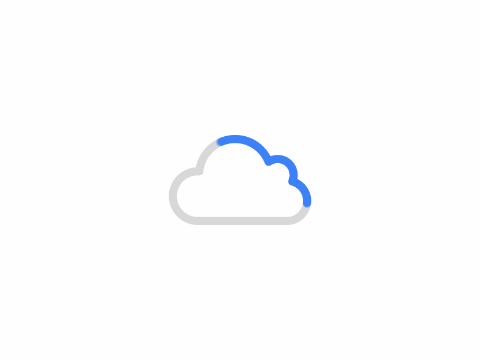
In some cases, you will also see a warning if you use Edge Caching. This is because it doesn’t support “narrow-scope” geolocation cache variations — near borders, for example. Instead, the cache is saved in each Point of Presence (PoP) location based on where the first visitor to the page lives.
Once you choose a geolocation setting and click the Enable button, MyKinsta will set everything up behind the scenes. After a few moments, you’ll see the Tools panel update with new options:
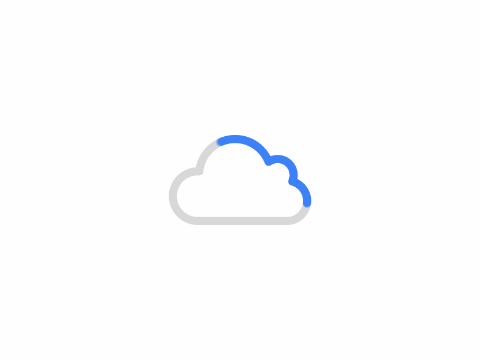
You have the option to disable geolocation under the “kebab” menu. Clicking Modify simply brings the geolocation choice modal dialog back up for you to tweak. Finally, if you want to enable geoblocking — which restricts access to your site based on the location — the Kinsta support team can do this for you, as it’s not available through the MyKinsta dashboard.
Building location-aware applications: practical examples
You have practically all of the tools and functionality available to you through NGINX and MyKinsta’s implementations of geolocation. This means you have plenty of scope to use both code snippets and Kinsta’s built-in capabilities to set up a solution to your needs.
For a no-code solution, Kinsta’s redirection functionality will be ideal.
Geographic-based redirects
One of the key facets of Kinsta’s IP Geolocation tool is how you can apply location-based conditions through sophisticated traffic routing.
If you were to use code for a setup like this, it would need some serious work:
class GeographicRedirects {
public function __construct() {
add_action('template_redirect', [$this, 'handle_redirects']);
}
public function handle_redirects() {
$country_code = $_SERVER['GEOIP_COUNTRY_CODE'] ?? null;
if ($country_code) {
$redirect_url = $this->get_redirect_url($country_code);
if ($redirect_url) {
wp_redirect($redirect_url, 301);
exit;
}
}
}
private function get_redirect_url($country_code) {
$redirects = [
'DE' => 'https://de.example.com',
'FR' => 'https://fr.example.com',
'ES' => 'https://es.example.com'
];
return $redirects[$country_code] ?? null;
}
}
new GeographicRedirects();
Instead, MyKinsta lets you use the Redirect panel, which includes fields for choosing a country and city.
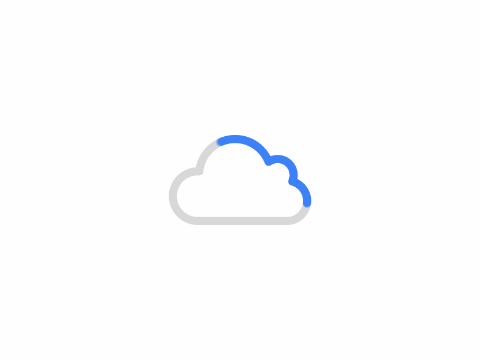
This is a powerful way to use Kinsta’s geolocation alongside redirect rules. You simply fill out the URLs you want to redirect from and to, choose a domain, select a country and city, and define the status code. Once you click the Add Redirect Rule button, Kinsta will apply it to your NGINX configuration.
Interactive map integration
A dynamic store locator will show the visitor’s location and any of your nearby stores. This will be ideal if you run a number of branches, especially if they are located across a wide expanse:
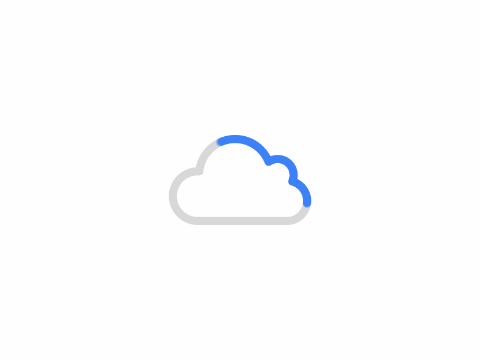
You can create a similar interactive experience using Kinsta’s IP Geolocation variables and the Google Maps API. To do so, you need a Google Maps API key and a basic understanding of the Google Maps JavaScript API. Also, you will need the location data of every store you run. The data can come from a custom post type or database in this instance.
For the implementation, it’s typical practice to add the code to your theme’s functions.php
file or a custom plugin. Once you set up your Google Maps API key, you can set up the store location data structure:
// Register the store locations post type
add_action('init', function() {
register_post_type('store_location', [
'public' => true,
'label' => 'Store Locations',
'supports' => ['title', 'editor', 'custom-fields'],
'show_in_rest' => true
]);
// Register custom fields for location data
register_meta('post', 'latitude', [
'type' => 'number',
'single' => true,
'show_in_rest' => true
]);
register_meta('post', 'longitude', [
'type' => 'number',
'single' => true,
'show_in_rest' => true
]);
});
To import your store locations, you can use the WordPress admin interface, create a custom post type, add the latitude and longitude into custom fields, add store details to your content, or even carry out a programmatic import. For example:
function import_store_locations($stores) {
foreach ($stores as $store) {
$post_id = wp_insert_post([
'post_type' => 'store_location',
'post_title' => sanitize_text_field($store['name']),
'post_status' => 'publish'
]);
if (!is_wp_error($post_id)) {
update_post_meta($post_id, 'latitude', floatval($store['lat']));
update_post_meta($post_id, 'longitude', floatval($store['lng']));
update_post_meta($post_id, 'address', sanitize_text_field($store['address']));
update_post_meta($post_id, 'phone', sanitize_text_field($store['phone']));
}
}
}
// Example usage:
$stores = [
[
'name' => 'Downtown Store',
'lat' => 40.7128,
'lng' => -74.0060,
'address' => '123 Main St',
'phone' => '(555) 123-4567'
]
// Add more stores...
];
import_store_locations($stores);
Implementing the locator will take a few lines of code and the exposed Kinsta variables:
class StoreLocator {
private $visitor_location;
private $google_maps_key;
public function __construct($google_maps_key) {
$this->google_maps_key = $google_maps_key;
$this->visitor_location = $this->get_visitor_location();
add_action('wp_enqueue_scripts', [$this, 'enqueue_maps_scripts']);
}
private function get_visitor_location() {
// Use Kinsta's geolocation data
if (isset($_SERVER['GEOIP_LATITUDE'], $_SERVER['GEOIP_LONGITUDE'])) {
return [
'lat' => floatval($_SERVER['GEOIP_LATITUDE']),
'lng' => floatval($_SERVER['GEOIP_LONGITUDE'])
];
}
// Fallback to country center
if (isset($_SERVER['GEOIP_COUNTRY_CODE'])) {
return $this->get_country_center($_SERVER['GEOIP_COUNTRY_CODE']);
}
// Default to New York
return ['lat' => 40.7128, 'lng' => -74.0060];
}
private function get_nearby_stores($radius = 50) {
return get_posts([
'post_type' => 'store_location',
'posts_per_page' => 10,
'meta_query' => [
[
'key' => 'latitude',
'value' => [
$this->visitor_location['lat'] - ($radius / 111),
$this->visitor_location['lat'] + ($radius / 111)
],
'type' => 'NUMERIC',
'compare' => 'BETWEEN'
]
]
]);
}
…
From here, you can add the map to your template using $store_locator->render_map()
.
Dynamic content delivery
Location-specific content, pricing, and promotions are bread-and-butter applications that rely on geolocation. Location-aware content delivery enables you to customize your site’s content, pricing, and promotions based on visitor location.
To implement this for your projects, you need the content variations for the different regions you want to target. From there, you can start to create a reliable way to handle location data and generate cache keys. This ensures efficient content delivery while maintaining location awareness:
private function get_location_context() {
// Create unique cache keys based on location data
$context = [
'country' => $_SERVER['GEOIP_COUNTRY_CODE'] ?? null,
'region' => $_SERVER['GEOIP_REGION'] ?? null,
'locale' => get_locale()
];
// Add currency and timezone data if needed
if ($this->requires_currency_handling) {
$context['currency'] = $this->get_country_currency($context['country']);
}
return $context;
}
This provides the foundation for any location-based decision and for your content processing system. This handles both pricing and regional content variations:
private function process_dynamic_content($content, $context) {
// Handle pricing with currency conversion
if (strpos($content, '{price:') !== false) {
$content = preg_replace_callback(
'//{price:([0-9]+/.?[0-9]*)/}/',
fn($matches) => $this->format_price(
floatval($matches[1]),
$context['currency']
),
$content
);
}
// Process regional content blocks
if (strpos($content, '[region:') !== false) {
$content = preg_replace_callback(
'//[region:([^/]]+)/](.*?)/[//region/]/s',
function($matches) use ($context) {
$regions = array_map('trim', explode(',', $matches[1]));
return in_array($context['country'], $regions) ? $matches[2] : '';
},
$content
);
}
return $content;
}
This enables you to use simple markers in your content that automatically adapt to the visitor’s location. For example:
[region:US,CA]
<p>Free shipping on orders over {price:50}!</p>
[/region]
[region:GB,DE,FR]
<p>Free shipping on orders over {price:45}!</p>
[/region]
With a simple implementation in place, you can optimize it for performance. This is a step that you might overlook, but efficient caching can help you maintain performance with your location-aware content. Kinsta’s caching models are ideal for the job.
Form pre-population and validation
Creating location-aware forms involves handling different address formats, postal codes, and phone numbers across regions. As such, it’s important to define the validation and formatting rules for those different regions:
private $format_patterns = [
'US' => [
'postal' => [
'pattern' => '^(?=.{5,10}$)/d{5}(-/d{4})?$',
'transform' => fn($value) => strtoupper(trim($value))
],
'phone' => [
'pattern' => '^/+1[2-9]/d{2}[2-9]/d{2}/d{4}$',
'transform' => fn($value) => '+1' . preg_replace('//D/', '', $value)
]
],
'GB' => [
'postal' => [
'pattern' => '^(?=.{6,8}$)[A-Z]{1,2}[0-9][A-Z0-9]? ?[0-9][A-Z]{2}$',
'transform' => fn($value) => preg_replace(
'/^(.+?)([0-9][A-Z]{2})$/',
'$1 $2',
strtoupper(trim($value))
)
]
]
];
These patterns handle common variations in postal codes and phone numbers across different countries. However, different countries have varying address format requirements. You can use a systematic approach to handling these variations:
private function get_address_format($country_code) {
$formats = [
'US' => [
'required' => ['street', 'city', 'state', 'postal'],
'order' => ['street', 'street2', 'city', 'state', 'postal'],
'state_label' => 'State',
'state_type' => 'select'
],
'GB' => [
'required' => ['street', 'city', 'postal'],
'order' => ['street', 'street2', 'city', 'county', 'postal'],
'state_label' => 'County',
'state_type' => 'text'
]
];
return $formats[$country_code] ?? $formats['US'];
}
Next, look at field validation. You should implement validation that respects regional format requirements:
private function validate_field($field, $value, $country_code) {
if (!isset($this->format_patterns[$country_code][$field])) {
return true; // No specific validation needed
}
$pattern = $this->format_patterns[$country_code][$field]['pattern'];
$transform = $this->format_patterns[$country_code][$field]['transform'];
// Transform value before validation
$value = $transform($value);
return (bool)preg_match("/$pattern/", $value);
}
A small snippet brings all of this together:
// Validate a postal code
if (!$this->validate_field('postal', $_POST['postal_code'], 'GB')) {
$errors[] = 'Invalid postal code format';
}
This implementation will automatically adapt to the visitor’s location, handle regional variations in address formats, provide proper validation for location-specific fields, and maintain data integrity across different regions.
Summary
Implementing WordPress geolocation through Kinsta’s infrastructure lets you create powerful location-aware applications. Enabling Kinsta’s IP Geolocation tool means you get to leverage server-level, robust, performant, and user-friendly functionality. What’s more, it’s privacy-centric and compliant.
Do you have any questions about WordPress geolocation and implementing it for your projects? Let us know in the comments section below!
The post WordPress geolocation made simple: a developer’s guide appeared first on Kinsta®.
共有 0 条评论