粒子系统类
书名:代码本色:用编程模拟自然系统
作者:Daniel Shiffman
译者:周晗彬
ISBN:978-7-115-36947-5
目录
4.4 粒子系统类
1、粒子系统类
到目前为止,我们已经做了两件事情:
- 首先,完成了粒子类,用它描述单个粒子对象;
- 之后,学会了ArrayList的用法,掌握了如何用它管理粒子对象列表(随心所欲
地添加和删除列表中的对象)。 - 下面还要做一件重要事情,那就是用一个类描述由粒子对象组成的系统——粒子系统类。
通过它,我们可以将复杂的遍历逻辑从主程序中移除,也可以非常方便地加入其他粒子系统。
2、新特性
- 比如,加入一个粒子的原点,也就是粒子创建的初始位置,即粒子的发射点,这恰好符合粒子系统“发射器”的概念。
- 这个原点必须在粒子系统的构造函数中初始化。
3、粒子系统类ParticleSystem
ParticleSystem.pde
class ParticleSystem {
ArrayList particles;
PVector origin; // 这个粒子系统包含一个原点
ParticleSystem(PVector position) {
origin = position.get();
particles = new ArrayList();
}
void addParticle() {
particles.add(new Particle(origin));
}
void run() {
for (int i = particles.size()-1; i >= 0; i--) {
Particle p = particles.get(i);
p.run();
if (p.isDead()) {
particles.remove(i);
}
}
}
}
4、示例
示例代码4-3 单个粒子系统
ParticleSystem ps;
void setup() {
size(640,360);
ps = new ParticleSystem(new PVector(width/2,50));
}
void draw() {
background(255);
ps.addParticle();
ps.run();
}
Particle.pde
class Particle {
PVector position;
PVector velocity;
PVector acceleration;
float lifespan;
color c;
Particle(PVector l) {
acceleration = new PVector(0,0.05);
velocity = new PVector(random(-1,1),random(-2,0));
position = l.get();
lifespan = 255.0;
c = color(random(255),random(255),random(255));
}
void run() {
update();
display();
}
// Method to update position
void update() {
velocity.add(acceleration);
position.add(velocity);
lifespan -= 2.0;
}
// Method to display
void display() {
stroke(0,lifespan);
strokeWeight(2);
fill(c,lifespan);
ellipse(position.x,position.y,12,12);
}
// Is the particle still useful?
boolean isDead() {
if (lifespan < 0.0) {
return true;
} else {
return false;
}
}
}
5、运行结果
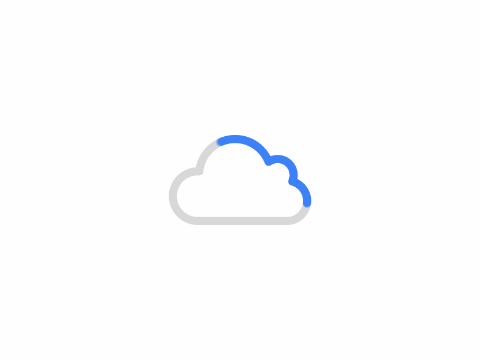
共有 0 条评论