(4) python 文件和异常
(一)文件操作
文件操作是非常重要的一部分,不管是网站建设中还是在深度学习中,难免会碰到需要对文件进行操作的时候,所以python中的文件操作是必不可少的。我们来简单看一下。
(1)文件的打开和关闭
使用open(文路径, 打开模式)
函数打开一个文件
使用write
对文件进行写入
使用read
对文件进行读取
使用seek
调整读写指针的位置,即从哪儿开始读或写
主要是熟悉几个函数的使用即可。
文件打开模式:
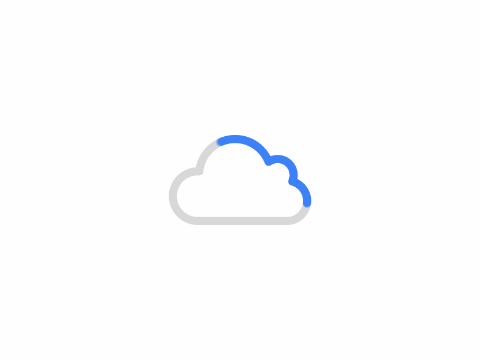
下面我们使用代码实现一下,使用代码创建一个文件1.txt
,写入“小黄不头秃”,并读取打印。
我们通常会使用到os模块来辅助文件操作.
使用with语句能够自动帮我们关闭打开的文件,可以不需要手动调用file.close().
import os
path = "./test/"
file_name = "1.txt"
if not os.path.exists(path):
# 如果该路径不存在
os.mkdir(path) # 创建该文件夹
# 使用w+模式打开文件,如果文件不存在则自动创建一个文件,编码使用UTF-8否则中文乱码
file = open(path+file_name, 'w+',encoding='utf-8')
file.write("小黄不头秃") # 写入
file.seek(0) # 将读写指针置零
print(file.read()) # 小黄不头秃 读取
file.close() # 关闭文件
使用os模块删除文件.
if os.path.exists(path+file_name): # 判断路径是否存在
os.remove(path+file_name)# 删除文件
print("file is deleted successfully")
else:
print("file is not exsist")
一个小demo,在test文件夹下创建四个文件,分别为1.txt
,2.txt
,3.csv
,4.word
,一共三类文件,将三类文件根据后缀名分别建立文件夹,并将对应后缀的文件复制到对应文件夹下。
# 先在test文件夹下创建四个文件,均写入'a'
craete_file_list = ["1.txt","2.txt","3.csv","4.word"]
path = "./test/"
if not os.path.exists(path):
os.mkdir(path)
for file in craete_file_list:
with open(path+file,'w+',encoding='utf-8') as f:
f.write('a')
f.close()
# 第一步:先获取所有文件的名字,除去文件夹,同时获取后缀名
path = './test/'
file_list = []
file_end = set()
for file in os.listdir(path):
if not os.path.isdir(path+file):
file_list.append(file)
end = file[file.rfind('.')+1:]
file_end.add(end)
# 第二步:创建对应的文件夹
for dir in file_end:
if not os.path.exists(path+dir):
os.mkdir(path+dir)
# 第三步复制文件到对应文件夹
for file in file_list:
src_path = path+file
des_path = path+file[file.rfind('.')+1:]+'/'+file
with open(src_path,'r+') as f:
content = f.read()
des_f = open(des_path,'w+',encoding='utf-8')
des_f.write(content)
f.close()
des_f.close()
(二)异常
(1)捕获异常
捕获异常使用try 执行代码 except 发生异常时执行的代码 else 没有异常时执行的代码 finally 不管有没有异常都会执行的代码
这样的代码结构。
# a = 1/0 # ZeroDivisionError: division by zero
try:
a = 1/0
print("123")
except Exception as e:
print("代码有错误!")
print("Error: ", e ,".")
except :
print("还有错误!")
else:
print("代码没有错误!")
finally:
print("代码执行完毕!")
(2)自定义异常
自定义异常类,用于处理一些特殊的场景,我们自己定义异常。
repr() 函数将对象转化为供解释器读取的形式。
class MyError(Exception):
def __init__(self, value) -> None:
self.value = value
def __str__(self) -> str:
# return repr("Please attention, something wrong in your code! the error is arround : {}".format(self.value))
return "Please attention, something wrong in your code! the error is arround : {}".format(self.value)
try:
y = -1
if y <= 0:
raise MyError(y)
except Exception as e:
print("Error: ",e)
共有 0 条评论