哈希/求和-三数求和
题目 LeetCode15
给你一个包含 n 个整数的数组 nums,判断 nums 中是否存在三个元素 a,b,c ,使得 a + b + c = 0 ?请你找出所有和为 0 且不重复的三元组。
示例 1:
输入:nums = [-1,0,1,2,-1,-4]
输出:[[-1,-1,2],[-1,0,1]]
解题思路
思路1:暴力求解,3 层循环。时间复杂度 O(nnn)
思路2:2 层循环,第 1 层循环遍历数组,作为 target,第二层循环参考两数求和的逻辑。
// 待优化的代码
public static List> threeSum(int[] nums) {
List> finalList = new ArrayList<>();
for (int i = 0; i < nums.length; i++) {
int target = -nums[i];
Set tempSet = new HashSet<>();
List tempList = new ArrayList<>();
// 遍历数组 nums
for (int j = 0; j < nums.length; j++) {
if(j == i){
continue;
}
// 每个值都判断 tempSet 中是否存在 target-nums[i]
if (tempSet.contains(target - nums[j])) {
tempList.add(nums[j]);
tempList.add(target - nums[j]);
tempList.add(nums[i]);
boolean flag = true;
// 判断 finalList 是否包含 tempList
for (List list : finalList) {
if(tempList.containsAll(list) && list.containsAll(tempList)){
flag = false;
break;
}
}
if(flag){
finalList.add(tempList);
}
tempList = new ArrayList<>();
}else{
// 如果不存在则将当前的 nums[i]存入 tempList 中,继续遍历直到找到为止
tempSet.add(nums[j]);
}
}
}
return finalList;
}
思路3:先排序后查找,时间复杂度 O(n*n)
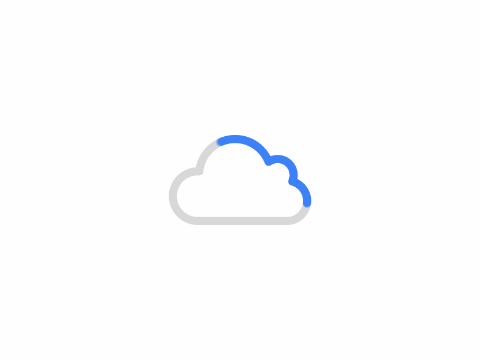
79467cc6d1844a55b65e5912320650b5.jpeg
class Solution {
public static List> threeSum(int[] nums) {
List> list = new ArrayList();
int len = nums.length;
if(nums == null || len < 3) return list;
Arrays.sort(nums); // 排序
for (int i = 0; i < len ; i++) {
if(nums[i] > 0) break; // 如果当前数字大于0,则三数之和一定大于0,所以结束循环
if(i > 0 && nums[i] == nums[i-1]) continue; // 则说明该数字重复,会导致结果重复,所以应该跳过
int L = i+1;//指针从第二个数开始移动
int R = len-1;
while(L < R){
int sum = nums[i] + nums[L] + nums[R];
if(sum == 0){
list.add(Arrays.asList(nums[i],nums[L],nums[R]));
while (L 0) R--;
}
}
return list;
}
}
共有 0 条评论